In this tutorial, you are going to learn the following topics.
Open the command line/terminal and run the following command
Run the following command to browse to the newly created project directory ci-app
You will get the following page
If you can see the above page then congratulations, you have successfully installed CodeIgniter.
As you can read from the above web page, the page displayed above is rendered by the view located in application/views/welcome_message.php and the responsible controller is located in application/controllers/Welcome.php
Let’s start with the home page route
Open the routes file as indicated by the path below
Open the following file
application/controllers/Welcome.php
You should be able to see the following code
Create a new file in application/views/home.php
Add the following code to home.php
The above HTML code loads Burma SSS framework and font from CDN network create a very basic HTML document. It applies very simple CSS rule from Burma CSS framework.
Open the following URL in your browser: http://localhost:3000/
You should be able to see the following
Great, we have just successfully modified the home page. Moving on, let’s define our route. Let’s assume our application also needs to be able to show the about us page.
Add the following route
Open application/controllers/Welcome.php
Add the following method
Create a new file about_us.php in application/views/about_us.php
Add the following code
You will see the following page
If you are able to see the above page then congratulations, you have successfully created a simple application in CodeIgniter.
- Routing – routing is responsible for responding to URL requests. Routing matches the URL to the pre-defined routes. If no route match is found then, CodeIgniter throws a page not found an exception.
- Controllers – routes are linked to controllers. Controllers glue the models and views together. The request for data/business logic from the model and return the results in the presentation of the view. Once a URL has been matched to a route, it is forwarded to a controller’s public function. This function interacts with the data source, business logic and returns the view that displays the results.
- Views – views are responsible for presentation. A view is usually a combination of HTML, CSS, and JavaScript. This is the part is responsible for displaying the web page to the user. Typically, the data displayed is usually retrieved from the database or any other available data sources.
How to create a new CodeIgniter project
We will use Composer to create a new project. I will be using the PHP built-in server, so it’s not necessary to have extra software such as Apache. In this tutorial, we are using the Windows operating system. Therefore, we have created a Sites folder on drive C. You can use any directory that is suitable for you.Open the command line/terminal and run the following command
HERE,cd C:\Sites
We will now create a CodeIgniter project using Composer. Run the following command
composer create-project CodeIgniter/framework ci-app
- The above command creates a new CodeIgniter project version 3 using the latest stable release i.e., 3.1.9 into a directory ci-app.
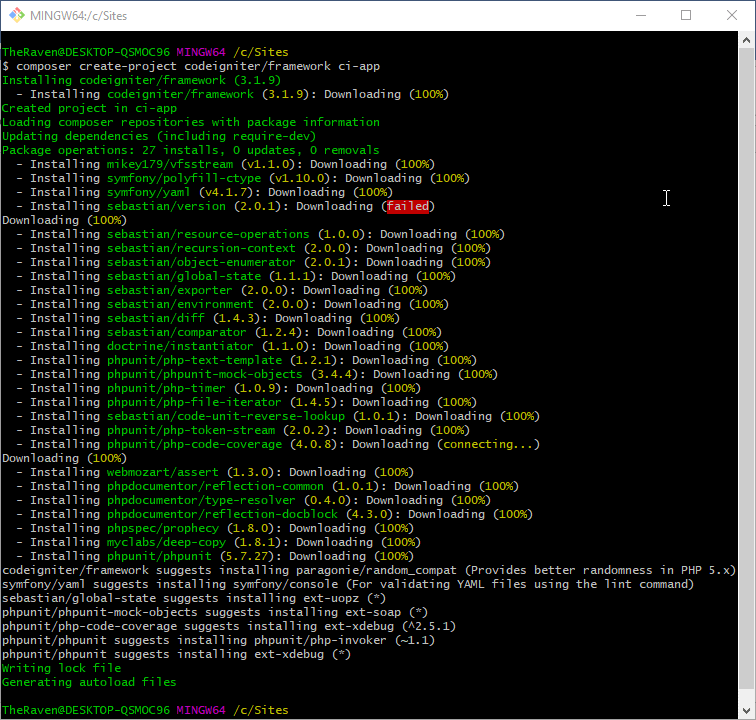
Run the following command to browse to the newly created project directory ci-app
HERE,cd ci-app
Let’s now start the PHP built-in web server
php -S localhost:3000
- The above command starts the built-in PHP server running on port 3000.
You will get the following page
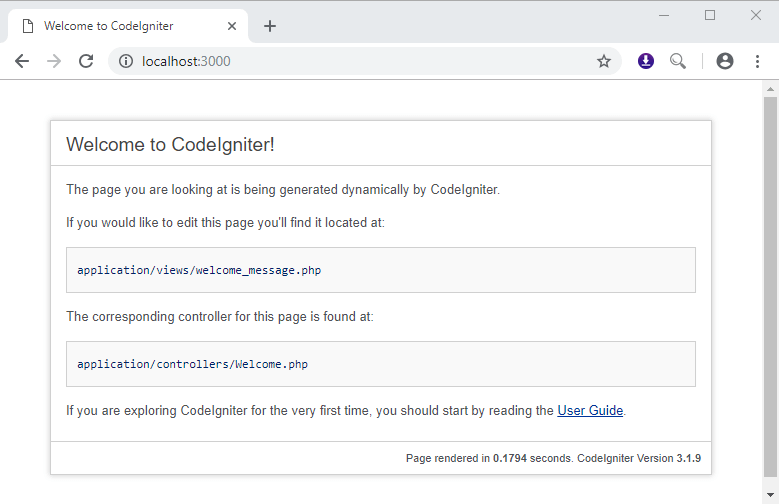
If you can see the above page then congratulations, you have successfully installed CodeIgniter.
As you can read from the above web page, the page displayed above is rendered by the view located in application/views/welcome_message.php and the responsible controller is located in application/controllers/Welcome.php
CodeIgniter Routing
For now, our application has only a single URL which is the home page. In this section, we will customize the home section. We will create some new URLs that will respond to the different requests.Let’s start with the home page route
Open the routes file as indicated by the path below
HERE,application/config/routes.php
You should be able to see the following content
$route['default_controller'] = 'welcome';
$route['404_override'] = '';
$route['translate_uri_dashes'] = FALSE;
- $route[‘default_controller’] = ‘welcome’; defines the default controller that responds to the URI requests
- $route[‘404_override’] = ”; this route allows you to define a custom route for 404 errors. A 404 error occurs when a page is not found. CodeIgniter has a default handler for the error but you can define your own if you so wish.
- $route[‘translate_uri_dashes’] = FALSE; this option allows you to translate dashes to underscores. We will talk about this option when we look at how routes work in CodeIgniter.
Open the following file
application/controllers/Welcome.php
You should be able to see the following code
HERE,<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Welcome extends CI_Controller {
public function index()
{
$this->load->view('welcome_message');
}
}
- Defined (‘BASEPATH’) OR exit(‘No direct script access allowed’); protects against directly accessing the controller class without passing through the index.php file. Remember, in MVC paradigm all requests have a single entry point, and for CodeIgniter, its index.php. This code blocks all requests that do not come through index.php
- class Welcome extends CI_Controller {…} defines a class Welcome that extends the parent class CI_Controller
- public function index() defines a public function that is called by default when you open the homepage
- $this->load->view(‘welcome_message’); this line loads the view welcome_message. The file welcome_message is located in the directory application/views/welcome_message.php
Create a new file in application/views/home.php
Add the following code to home.php
HERE,<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Hello CodeIgniter!</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bulma/0.7.2/css/bulma.min.css">
<script defer src="https://use.fontawesome.com/releases/v5.3.1/js/all.js"></script>
</head>
<body>
<section class="section">
<div class="container">
<h1 class="title">
CodeIgniter Hello World
</h1>
</div>
</section>
</body>
</html>
The above HTML code loads Burma SSS framework and font from CDN network create a very basic HTML document. It applies very simple CSS rule from Burma CSS framework.
Open the following URL in your browser: http://localhost:3000/
You should be able to see the following

Great, we have just successfully modified the home page. Moving on, let’s define our route. Let’s assume our application also needs to be able to show the about us page.
Create a Route
Open the routes file application/config.routes.phpAdd the following route
HERE,$route['about-us'] = 'welcome/about_us';
- When a visitor visits the URL /about-us, we are instructing CodeIgniter to look for a controller Welcome and execute the method about_us.
Create a Controller
Let’s now define the controller method about usOpen application/controllers/Welcome.php
Add the following method
HERE,public function about_us(){
$this->load->view('about_us');
}
- The above code defines a function about_us and loads a view about_us.
Create a View
Let’s now create the view that we just referenced in the above sectionCreate a new file about_us.php in application/views/about_us.php
Add the following code
We are good to go, open the following URL in your web browser: http://localhost:3000/index.php/about-us<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>About CodeIgniter!</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bulma/0.7.2/css/bulma.min.css">
<script defer src="https://use.fontawesome.com/releases/v5.3.1/js/all.js"></script>
</head>
<body>
<section class="section">
<div class="container">
<h1 class="title">
About us yap...
</h1>
</div>
</section>
</body>
</html>
You will see the following page
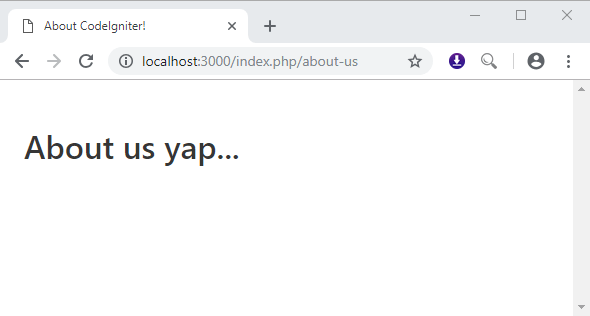
If you are able to see the above page then congratulations, you have successfully created a simple application in CodeIgniter.