alexanderm
New member
Hello everyone,
I have a task in which I would like to implement the graphical visualisation of a quadratic equation in the interval x =[-10,10] and - if any exist - the corresponding zeros, using CanvasRenderingContext2D methods.
To convert the coordinates into pixel coordinates within the canvas: the coordinates into pixel coordinates within the canvas I would use following functions:
var toCanvasX = function ( x ) {
return (x + (max-min ) / 2 ) * canvas.width /( max - min );
}
var toCanvasY = function ( y ) {
return canvas.height - (y + (max-min ) / 2 ) * canvas.height / ( max - min);
}
The graph should look like this:
How can I solve it?
Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<h1>Solver of Quadratic Equations</h1>
<script>
var a, b, c;
var output;
function check() {
a = document.forms["input_form"]["anumber"].value;
b = document.forms["input_form"]["bnumber"].value;
c = document.forms["input_form"]["cnumber"].value;
if (a == 0) {
output = "a cannot equal zero!";
} else if (isNaN(a)) {
output = "a has to be a number!";
} else if (isNaN(b)) {
output = "b has to be a number!";
} else if (isNaN(c)) {
output = "c has to be a number!";
} else {
var x1 = (-b - Math.sqrt(Math.pow(b, 2) - 4 * a * c)) / (2 * a);
var x2 = (-b + Math.sqrt(Math.pow(b, 2) - 4 * a * c)) / (2 * a);
output = "The polynomial <strong>" + (a == 1 ? "" : a) + "x\u00B2 + " + (b == 1 ? "" : b) + "x + " + c + " = 0</strong> has two zeros x1=" + x1 + "," + " " + "x2=" + x2;
}
document.getElementById("output").innerHTML = output;
}
</script>
</head>
<body>
This programme calculates zeros of quadratic polynomials of the form ax² + bx + c and graphically displays the solution in the interval x ∈ [-10,10].
<br><br>
<form name="input_form" action="javascript:check();">
a: <input type="text" name="anumber" required>
b: <input type="text" name="bnumber" required>
c: <input type="text" name="cnumber" required>
<br><br>
<input type="submit" value="Calculate zeros">
</form>
<p id="output"/>
</body>
</html>
I have a task in which I would like to implement the graphical visualisation of a quadratic equation in the interval x =[-10,10] and - if any exist - the corresponding zeros, using CanvasRenderingContext2D methods.
To convert the coordinates into pixel coordinates within the canvas: the coordinates into pixel coordinates within the canvas I would use following functions:
var toCanvasX = function ( x ) {
return (x + (max-min ) / 2 ) * canvas.width /( max - min );
}
var toCanvasY = function ( y ) {
return canvas.height - (y + (max-min ) / 2 ) * canvas.height / ( max - min);
}
The graph should look like this:
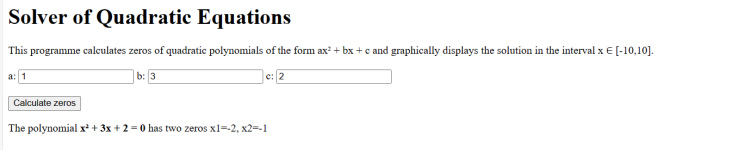
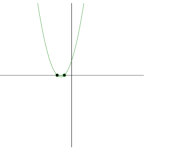
How can I solve it?
Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<h1>Solver of Quadratic Equations</h1>
<script>
var a, b, c;
var output;
function check() {
a = document.forms["input_form"]["anumber"].value;
b = document.forms["input_form"]["bnumber"].value;
c = document.forms["input_form"]["cnumber"].value;
if (a == 0) {
output = "a cannot equal zero!";
} else if (isNaN(a)) {
output = "a has to be a number!";
} else if (isNaN(b)) {
output = "b has to be a number!";
} else if (isNaN(c)) {
output = "c has to be a number!";
} else {
var x1 = (-b - Math.sqrt(Math.pow(b, 2) - 4 * a * c)) / (2 * a);
var x2 = (-b + Math.sqrt(Math.pow(b, 2) - 4 * a * c)) / (2 * a);
output = "The polynomial <strong>" + (a == 1 ? "" : a) + "x\u00B2 + " + (b == 1 ? "" : b) + "x + " + c + " = 0</strong> has two zeros x1=" + x1 + "," + " " + "x2=" + x2;
}
document.getElementById("output").innerHTML = output;
}
</script>
</head>
<body>
This programme calculates zeros of quadratic polynomials of the form ax² + bx + c and graphically displays the solution in the interval x ∈ [-10,10].
<br><br>
<form name="input_form" action="javascript:check();">
a: <input type="text" name="anumber" required>
b: <input type="text" name="bnumber" required>
c: <input type="text" name="cnumber" required>
<br><br>
<input type="submit" value="Calculate zeros">
</form>
<p id="output"/>
</body>
</html>